cylindrical_plug
#
This creates a cylinder which can be used to define the outer regions of a tomogram.
import matplotlib.pyplot as plt
import numpy as np
import porespy as ps
import inspect
[17:46:00] ERROR PARDISO solver not installed, run `pip install pypardiso`. Otherwise, _workspace.py:56 simulations will be slow. Apple M chips not supported.
The arguments and defaults for this function can be listed as follows:
inspect.signature(ps.generators.cylindrical_plug)
<Signature (shape, r=None, axis=2)>
shape
#
This should be the same shape as the image which is being studied. Note that if the image does not have odd valued dimensions then the cylinder will not be centered.
im = ps.generators.blobs(shape=[15, 15, 15], porosity=0.6)
cyl = ps.generators.cylindrical_plug(shape=im.shape)
ax = plt.figure(figsize=[6, 6]).add_subplot(projection='3d')
ax.voxels(cyl, edgecolor='k', linewidth=.25);
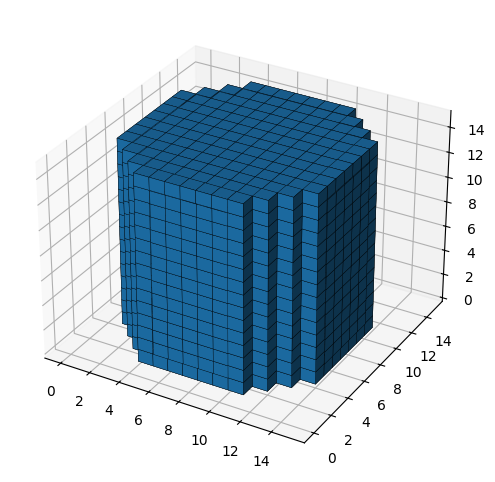
Or to generate a 2D disk
cyl = ps.generators.cylindrical_plug(shape=[21, 21])
plt.imshow(cyl, origin='lower', interpolation='none')
plt.axis(False);
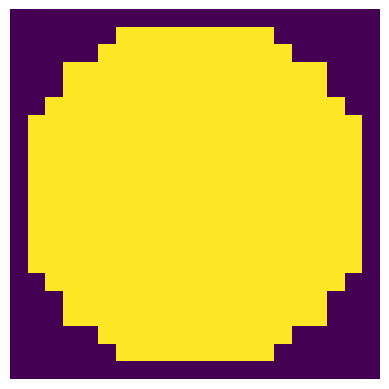
r
#
The radius of the plug. By default it will fill the image, but it can be smaller or larger than the image too.
fig, ax = plt.subplots(1, 2, figsize=[12, 6])
r = 5
cyl = ps.generators.cylindrical_plug(shape=im.shape, r=r)
ax[0].imshow(cyl[..., 10], interpolation='none', origin='lower')
ax[0].axis(False)
r = 8
cyl = ps.generators.cylindrical_plug(shape=im.shape, r=r)
ax[1].imshow(cyl[..., 10], interpolation='none', origin='lower')
ax[1].axis(False);
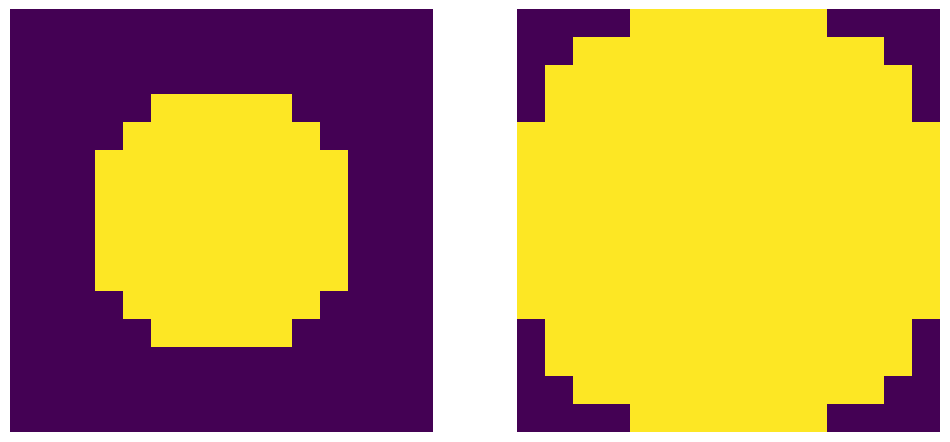
axis
#
The orientation of the plug can be aligned with any axis:
fig, (ax1, ax2, ax3) = plt.subplots(ncols=3, subplot_kw={'projection': '3d'}, figsize=[15, 5])
cyl = ps.generators.cylindrical_plug(shape=im.shape, axis=0)
ax1.voxels(cyl, edgecolor='k', linewidth=.25)
cyl = ps.generators.cylindrical_plug(shape=im.shape, axis=1)
ax2.voxels(cyl, edgecolor='k', linewidth=.25)
cyl = ps.generators.cylindrical_plug(shape=im.shape, axis=2)
ax3.voxels(cyl, edgecolor='k', linewidth=.25);
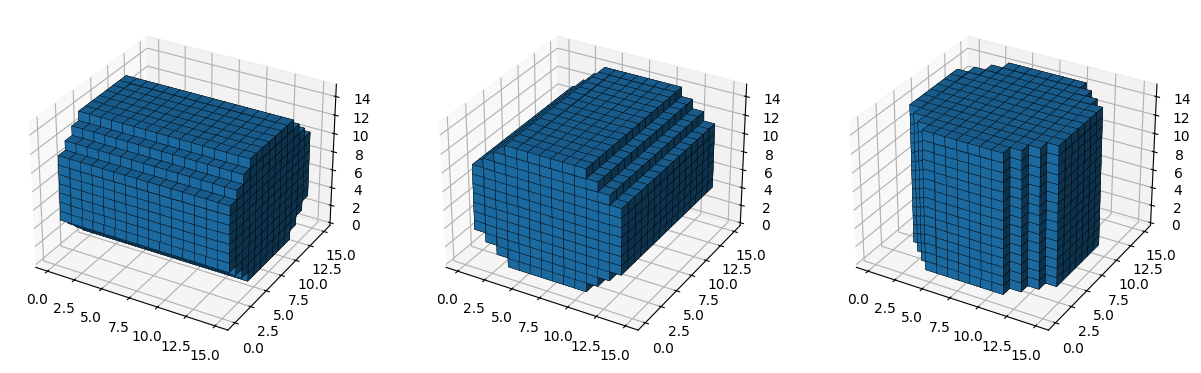