bundle_of_tubes
#
Create a 3D image of a bundle of tubes, in the form of a rectangular plate with randomly sized holes through it.
import inspect
import matplotlib.pyplot as plt
import numpy as np
import porespy as ps
inspect.signature(ps.generators.bundle_of_tubes)
<Signature (shape: List[int], spacing: int, distribution=None, smooth: bool = True, seed: int = None)>
spacing
#
Controls how far apart each pore is. Note that this limits the maximum size of each pore since they are prevented from overlapping.
fig, ax = plt.subplots(1, 2, figsize=[8, 4])
np.random.seed(10)
shape = [300, 300]
spacing = 10
im = ps.generators.bundle_of_tubes(shape=shape, spacing=spacing)
ax[0].imshow(im, origin='lower', interpolation='none')
ax[0].axis(False)
spacing = 15
im = ps.generators.bundle_of_tubes(shape=shape, spacing=spacing)
ax[1].imshow(im, origin='lower', interpolation='none')
ax[1].axis(False);
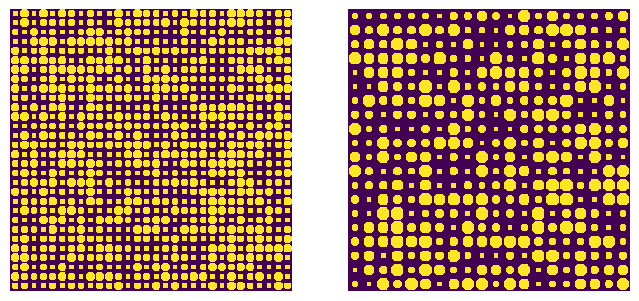
distribution
#
The default size distribution is uniform (i.e. random) with sizes ranging between 3 and spacing - 1
. To use a different distribution it can specify using a predefined scipy.stats
object. If care is not taken to ensure the distribution only returns values between 3 and spacing - 1
then the value are clipped accordingly.
import scipy.stats as spst
fig, ax = plt.subplots(1, 2, figsize=[8, 4])
dst = spst.norm(loc=8, scale=1)
im = ps.generators.bundle_of_tubes(shape=shape, spacing=spacing, distribution=dst)
ax[0].imshow(im, origin='lower', interpolation='none')
ax[0].axis(False)
dst = spst.norm(loc=10, scale=4)
im = ps.generators.bundle_of_tubes(shape=shape, spacing=spacing, distribution=dst)
ax[1].imshow(im, origin='lower', interpolation='none')
ax[1].axis(False);
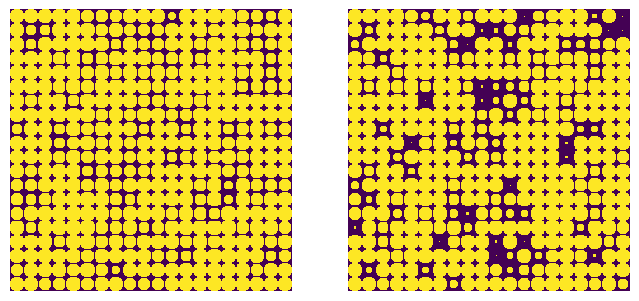