chord_counts
¶
Calculates the length of each chord in the input image that contains chords drawn in the void space. It works by labeling each chord and using Scikit image regionprops to measure the length of each chord (number of pixels in the chord).
import matplotlib.pyplot as plt
import numpy as np
import porespy as ps
import inspect
inspect.signature(ps.metrics.chord_counts)
<Signature (im)>
im
¶
The input image ontaining chords drawn in the void space. This image can be generated by implementing apply_chords
filter on a binary image. We can use blobs
generator to generate a test image and draw chords in void space (in x direction by default) using apply_chords
.
np.random.seed(10)
im = ps.generators.blobs(shape=[500, 500])
im = ps.filters.apply_chords(im)
fig, ax = plt.subplots(1, 1, figsize=[4, 4])
ax.imshow(im, origin='lower', interpolation='none')
ax.axis(False);
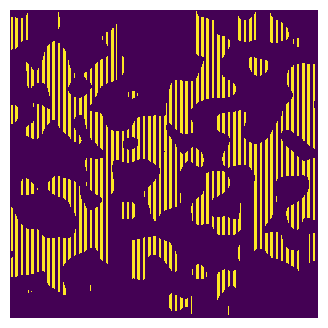
Now the test image is ready to be passed to chord_counts
. The method returns a 1D array. Each element in the array corresponds to the length of each chord. A histogram of the chord length array can be plotted:
chord_length = ps.metrics.chord_counts(im=im)
fig, ax = plt.subplots(1, 1, figsize=[4, 4])
ax.hist(chord_length, bins=100)
plt.xlabel('chord length along x axis')
plt.ylabel('frequency');
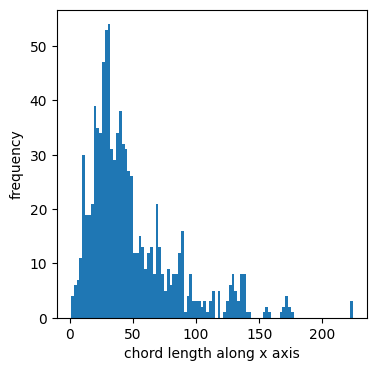
An alternative to the histogram, we can use numpy.bincount
that gives the number of chords of each length and can be plotted using plt.plot
. The resulting plot is similar to the histogram plot with a high number of bins:
fig, ax = plt.subplots(1, 1, figsize=[4, 4])
ax.plot(np.bincount(chord_length))
plt.xlabel('chord length')
plt.ylabel('the number of chords of each length');
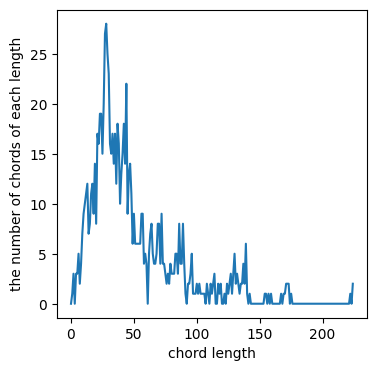
np.bincount(chord_length)
array([ 0, 1, 3, 0, 3, 3, 5, 2, 4, 7, 9, 10, 11, 12, 7, 8, 11,
12, 9, 14, 8, 17, 16, 19, 19, 15, 20, 27, 28, 25, 23, 16, 15, 17,
14, 17, 12, 18, 16, 10, 13, 15, 18, 14, 22, 9, 13, 14, 11, 6, 9,
6, 6, 6, 6, 6, 9, 9, 4, 5, 4, 0, 5, 7, 8, 5, 4, 4,
5, 8, 8, 4, 9, 4, 4, 3, 2, 3, 2, 4, 3, 3, 3, 5, 5,
3, 8, 4, 4, 8, 4, 1, 0, 2, 2, 3, 5, 1, 1, 1, 2, 1,
2, 1, 1, 1, 1, 0, 2, 1, 0, 2, 1, 2, 3, 0, 0, 2, 1,
2, 0, 0, 1, 0, 2, 1, 2, 3, 1, 3, 5, 2, 3, 2, 1, 2,
2, 4, 2, 6, 1, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 1, 1, 0, 1, 0, 1, 0, 0, 0, 0, 0, 0, 0, 1, 0, 1,
1, 2, 2, 2, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 1, 0, 2], dtype=int64)